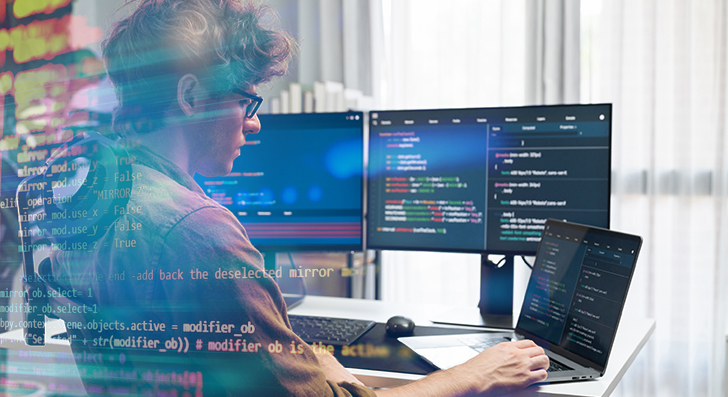
Scalability indicates your application can manage development—more buyers, far more info, and even more site visitors—with out breaking. To be a developer, constructing with scalability in mind will save time and anxiety later on. Here’s a transparent and useful guide to assist you to start off by Gustavo Woltmann.
Design for Scalability from the beginning
Scalability isn't a thing you bolt on afterwards—it should be aspect of one's approach from the beginning. Lots of programs are unsuccessful once they develop rapid simply because the first style can’t cope with the extra load. Being a developer, you need to Feel early regarding how your technique will behave stressed.
Begin by coming up with your architecture to become versatile. Stay clear of monolithic codebases exactly where anything is tightly connected. As a substitute, use modular style or microservices. These designs split your application into smaller, impartial sections. Each module or support can scale By itself with out impacting The full process.
Also, consider your database from day one particular. Will it have to have to handle a million people or just a hundred? Choose the proper variety—relational or NoSQL—based upon how your details will grow. Strategy for sharding, indexing, and backups early, Even though you don’t have to have them yet.
An additional essential level is in order to avoid hardcoding assumptions. Don’t produce code that only will work less than present-day disorders. Take into consideration what would transpire If the person foundation doubled tomorrow. Would your application crash? Would the database slow down?
Use design and style patterns that assistance scaling, like concept queues or celebration-driven techniques. These support your app deal with much more requests with out obtaining overloaded.
Whenever you Create with scalability in mind, you're not just preparing for fulfillment—you happen to be minimizing potential head aches. A well-prepared process is less complicated to keep up, adapt, and expand. It’s much better to prepare early than to rebuild afterwards.
Use the best Database
Choosing the ideal databases is really a crucial A part of building scalable apps. Not all databases are developed exactly the same, and utilizing the Mistaken one can gradual you down and even result in failures as your app grows.
Start out by knowing your data. Can it be extremely structured, like rows inside of a table? If yes, a relational databases like PostgreSQL or MySQL is an effective match. These are solid with relationships, transactions, and regularity. They also guidance scaling strategies like browse replicas, indexing, and partitioning to manage more website traffic and knowledge.
In case your facts is more versatile—like person activity logs, product or service catalogs, or documents—look at a NoSQL choice like MongoDB, Cassandra, or DynamoDB. NoSQL databases are superior at dealing with significant volumes of unstructured or semi-structured info and might scale horizontally more simply.
Also, consider your go through and produce patterns. Do you think you're accomplishing a great deal of reads with much less writes? Use caching and read replicas. Do you think you're managing a heavy compose load? Check into databases which can deal with substantial produce throughput, or even celebration-centered information storage techniques like Apache Kafka (for momentary details streams).
It’s also smart to Believe ahead. You may not need to have State-of-the-art scaling attributes now, but selecting a database that supports them signifies you received’t have to have to modify afterwards.
Use indexing to hurry up queries. Avoid unnecessary joins. Normalize or denormalize your information according to your obtain styles. And normally observe databases general performance when you mature.
To put it briefly, the right databases relies on your application’s structure, speed needs, And exactly how you hope it to mature. Choose time to select correctly—it’ll preserve plenty of problems later.
Improve Code and Queries
Quick code is key to scalability. As your application grows, just about every modest delay adds up. Improperly published code or unoptimized queries can decelerate efficiency and overload your method. That’s why it’s crucial to Construct effective logic from the beginning.
Start out by composing thoroughly clean, simple code. Stay clear of repeating logic and take away anything at all pointless. Don’t pick the most intricate Remedy if an easy one is effective. Maintain your functions brief, concentrated, and simple to test. Use profiling instruments to discover bottlenecks—places wherever your code will take way too lengthy to operate or makes use of too much memory.
Following, take a look at your databases queries. These frequently gradual issues down much more than the code by itself. Be certain Just about every query only asks for the information you actually need to have. Avoid Decide on *, which fetches everything, and alternatively select distinct fields. Use indexes to hurry up lookups. And stay away from accomplishing too many joins, Primarily across massive tables.
If you recognize a similar information currently being asked for again and again, use caching. Keep the effects temporarily making use of tools like Redis or Memcached which means you don’t should repeat expensive operations.
Also, batch your database functions any time you can. Instead of updating a row one by one, update them in groups. This cuts down on overhead and can make your application extra efficient.
Make sure to take a look at with large datasets. Code and queries that function fantastic with one hundred data could crash when they have to handle 1 million.
In brief, scalable apps are quickly apps. Keep the code limited, your queries lean, and use caching when desired. These steps help your application stay smooth and responsive, even as the load increases.
Leverage Load Balancing and Caching
As your app grows, it has to handle more users and much more website traffic. If anything goes by just one server, it can immediately turn into a bottleneck. That’s the place load balancing and caching can be found in. These two resources assist keep your application rapid, secure, and scalable.
Load balancing spreads incoming targeted visitors throughout a number of servers. As an alternative to a single server carrying out all of the function, the load balancer routes users to distinctive servers based upon availability. What this means is no single server receives overloaded. If just one server goes down, the load balancer can ship visitors to the Other individuals. Tools like Nginx, HAProxy, or cloud-centered alternatives from AWS and Google Cloud make this simple to set up.
Caching is about storing details briefly so it may be reused immediately. When people request a similar facts once more—like a product web site or possibly a profile—you don’t have to fetch it within the database every time. You could serve it within the cache.
There are 2 common forms of caching:
1. Server-aspect caching (like Redis or Memcached) shops facts in memory for rapidly access.
two. Client-aspect caching (like browser caching or CDN caching) stores static documents near the user.
Caching cuts down database load, enhances velocity, and can make your application a lot more economical.
Use caching for things that don’t improve typically. And normally ensure your cache is current when information does transform.
In short, load balancing and caching are basic but powerful equipment. Alongside one another, they help your app take care of more consumers, keep quickly, and Get well from problems. If you plan to increase, you would like each.
Use Cloud and Container Equipment
To develop scalable applications, you'll need equipment that permit your application grow effortlessly. That’s the place cloud platforms and containers can be found in. They give you versatility, lessen set up time, and make scaling A great deal smoother.
Cloud platforms like Amazon Web Providers (AWS), Google Cloud Platform (GCP), and Microsoft Azure Allow you to lease servers and expert services as you would like them. You don’t have to buy hardware or guess long term capacity. When site visitors will increase, you could add more resources with just a few clicks or automatically using auto-scaling. When traffic drops, you can scale down to save money.
These platforms also offer products and services like managed databases, storage, load balancing, and stability applications. You could center on making your application as opposed to taking care of infrastructure.
Containers are One more essential Device. A container packages your application and anything it ought to run—code, libraries, settings—into one device. This causes it to be simple to maneuver your application among environments, from your notebook to your cloud, without having surprises. Docker is the most well-liked Instrument for this.
Once your application makes use of multiple containers, applications like Kubernetes allow you to control them. Kubernetes handles deployment, scaling, and Restoration. If one particular element of your application crashes, it restarts it immediately.
Containers also enable it to be very easy to separate portions of your app into products and services. You could update or scale areas independently, that is perfect for efficiency and trustworthiness.
In a nutshell, using cloud and container equipment means you may scale quick, deploy quickly, and recover promptly when issues transpire. If you'd like your application to grow with no restrictions, start out utilizing these instruments early. They save time, lessen hazard, and enable you to keep centered on developing, not repairing.
Observe Every thing
When you don’t monitor your application, you gained’t know when points go wrong. Monitoring will help the thing is how your application is carrying out, location challenges early, and make much better choices as your application grows. It’s a vital part of creating scalable programs.
Get started read more by monitoring basic metrics like CPU usage, memory, disk Area, and response time. These inform you how your servers and expert services are accomplishing. Tools like Prometheus, Grafana, Datadog, or New Relic will help you acquire and visualize this knowledge.
Don’t just watch your servers—watch your application as well. Keep watch over just how long it requires for end users to load web pages, how often problems come about, and the place they come about. Logging instruments like ELK Stack (Elasticsearch, Logstash, Kibana) or Loggly may help you see what’s occurring within your code.
Put in place alerts for critical troubles. One example is, If the reaction time goes previously mentioned a limit or perhaps a services goes down, you need to get notified quickly. This aids you resolve problems fast, often right before people even detect.
Monitoring can also be useful after you make improvements. In case you deploy a fresh function and find out a spike in glitches or slowdowns, you'll be able to roll it back right before it brings about actual damage.
As your application grows, site visitors and information improve. Without the need of checking, you’ll miss indications of problems until it’s far too late. But with the best tools in position, you stay on top of things.
In brief, checking aids you keep the app responsible and scalable. It’s not nearly recognizing failures—it’s about comprehension your system and making certain it works properly, even under pressure.
Ultimate Views
Scalability isn’t just for large corporations. Even little applications need a robust Basis. By developing diligently, optimizing properly, and utilizing the right equipment, you can Construct applications that develop efficiently without the need of breaking under pressure. Start off small, Feel major, and build sensible.